For example, I need to change attribute's label and make it readonly on the fly. In my ViewObjectImpl class I have the following method:
public void setCustomHints() { AttributeDefImpl attr = ((AttributeDefImpl) findAttributeDef("DepartmentName")); attr.setProperty("customLabel", "The custom label"); attr.setProperty("readonly", Boolean.TRUE); }
The setCustomHints method is going to be executed on a commandButton's action. And the jspx page contains the following piece of code:
<af:inputText value="#{bindings.DepartmentName.inputValue}" label="#{bindings.DepartmentName.hints.customLabel}" readonly="#{bindings.DepartmentName.hints.readonly}" columns="#{bindings.DepartmentName.hints.displayWidth}" maximumLength="#{bindings.DepartmentName.hints.precision}" shortDesc="#{bindings.DepartmentName.hints.tooltip}" id="it4" partialTriggers="cb1"> <f:validator binding="#{bindings.DepartmentName.validator}"/> </af:inputText> <af:commandButton actionListener="#{bindings.setCustomHints.execute}" text="setCustomHints" disabled="#{!bindings.setCustomHints.enabled}" id="cb1"/>
Additionally, I set up default values for customLabel and readonly custom properties at the VO's definition page:
And finally, we've got the following result:
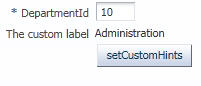
The sample application for this post is available.